解决思路:自定义一个拦截器,当有模糊查询时,模糊查询的关键字中包含有上述特殊字符时,在该特殊字符前添加\进行转义处理。
问题提出
使用MyBatis中的模糊查询时,当查询关键字中包括有_、\、%时,查询关键字失效。
问题分析
- 当like中包含时,查询仍为全部,即 like ‘%%’查询出来的结果与like ‘%%’一致,并不能查询出实际字段中包含有_特殊字符的结果条目
- like中包括%时,与1中相同
- like中包含\时,带入查询时,%%无法查询到包含字段中有\的条目
特殊字符 |
未处理 |
处理后 |
_ |
like ‘%_%’ |
like ‘%_%’ |
% |
like ‘%%%’ |
like ‘%%%’ |
\ |
like ‘%%‘ ` |
like ‘%%‘ |
ESCAPE ‘/‘必须加在SQL的最后。
like ‘%_%’效果与like concat(‘%’, ‘_‘, ‘%’)相同
问题解决
- 自定义拦截器方法类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; import com.keyidea.boss.utils.EscapeUtil; import org.apache.ibatis.executor.Executor; import org.apache.ibatis.mapping.BoundSql; import org.apache.ibatis.mapping.MappedStatement; import org.apache.ibatis.plugin.*; import org.apache.ibatis.session.ResultHandler; import org.apache.ibatis.session.RowBounds; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import java.util.HashMap; import java.util.HashSet; import java.util.Properties; import java.util.Set;
@Intercepts(@Signature(type = Executor.class, method = "query", args = {MappedStatement.class, Object.class, RowBounds.class, ResultHandler.class})) public class MyInterceptor implements Interceptor {
Logger LOGGER = LoggerFactory.getLogger(MyInterceptor.class);
@Override public Object intercept(Invocation invocation) throws Throwable { Object[] args = invocation.getArgs(); MappedStatement statement = (MappedStatement)args[0]; Object parameterObject = args[1]; BoundSql boundSql = statement.getBoundSql(parameterObject); String sql = boundSql.getSql(); modifyLikeSql(sql, parameterObject, boundSql); return invocation.proceed(); }
@Override public Object plugin(Object target) { return Plugin.wrap(target, this); }
@Override public void setProperties(Properties properties) {
}
@SuppressWarnings("unchecked") public static String modifyLikeSql(String sql, Object parameterObject, BoundSql boundSql) { if (parameterObject instanceof HashMap) { } else { return sql; } if (!sql.toLowerCase().contains(" like ") || !sql.toLowerCase().contains("?")) { return sql; } String[] strList = sql.split("\\?"); Set<String> keyNames = new HashSet<>(); for (int i = 0; i < strList.length; i++) { if (strList[i].toLowerCase().contains(" like ")) { String keyName = boundSql.getParameterMappings().get(i).getProperty(); keyNames.add(keyName); } } for (String keyName : keyNames) { HashMap parameter = (HashMap)parameterObject; if (keyName.contains("ew.paramNameValuePairs.") && sql.toLowerCase().contains(" like ?")) { QueryWrapper wrapper = (QueryWrapper)parameter.get("ew"); parameter = (HashMap)wrapper.getParamNameValuePairs();
String[] keyList = keyName.split("\\."); Object a = parameter.get(keyList[2]); if (a instanceof String && (a.toString().contains("_") || a.toString().contains("\\") || a.toString() .contains("%"))) { parameter.put(keyList[2], "%" + EscapeUtil.escapeChar(a.toString().substring(1, a.toString().length() - 1)) + "%"); } } else if (!keyName.contains("ew.paramNameValuePairs.") && sql.toLowerCase().contains(" like ?")) { Object a = parameter.get(keyName); if (a instanceof String && (a.toString().contains("_") || a.toString().contains("\\") || a.toString() .contains("%"))) { parameter.put(keyName, "%" + EscapeUtil.escapeChar(a.toString().substring(1, a.toString().length() - 1)) + "%"); } } else { Object a = parameter.get(keyName); if (a instanceof String && (a.toString().contains("_") || a.toString().contains("\\") || a.toString() .contains("%"))) { parameter.put(keyName, EscapeUtil.escapeChar(a.toString())); } } } return sql; } }
|
- 在配置类中添加自定义拦截器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79
|
import com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceBuilder; import com.baomidou.mybatisplus.autoconfigure.MybatisPlusProperties; import com.baomidou.mybatisplus.core.config.GlobalConfig; import com.baomidou.mybatisplus.extension.plugins.PaginationInterceptor; import com.baomidou.mybatisplus.extension.spring.MybatisSqlSessionFactoryBean; import org.apache.ibatis.plugin.Interceptor; import org.apache.ibatis.session.SqlSessionFactory; import org.mybatis.spring.annotation.MapperScan; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import org.springframework.jdbc.datasource.DataSourceTransactionManager; import javax.sql.DataSource; import java.util.ArrayList; import java.util.List;
@Configuration @MapperScan(basePackages = {"com.keyidea.boss.mapper"}, sqlSessionFactoryRef = "masterSqlSessionFactory") public class MybatisPlusConfig {
@Autowired private MybatisPlusProperties properties; @Bean public PaginationInterceptor paginationInterceptor() { PaginationInterceptor page = new PaginationInterceptor(); return page; } @Bean public MyInterceptor myInterceptor() { MyInterceptor sql = new MyInterceptor(); return sql; } @Bean(name = "masterDataSource") @ConfigurationProperties("spring.datasource") @Primary public DataSource masterDataSource() { return DruidDataSourceBuilder.create().build(); } @Bean(name = "masterSqlSessionFactory") @Primary public SqlSessionFactory masterSqlSessionFactory(@Qualifier("masterDataSource") DataSource dataSource, PaginationInterceptor paginationInterceptor, MyInterceptor myInterceptor) throws Exception { final MybatisSqlSessionFactoryBean sessionFactory = new MybatisSqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
if (this.properties.getConfigurationProperties() != null) { sessionFactory.setConfigurationProperties(this.properties.getConfigurationProperties()); } GlobalConfig globalConfig = this.properties.getGlobalConfig(); sessionFactory.setGlobalConfig(globalConfig); List<Interceptor> interceptors = new ArrayList<>(); interceptors.add(paginationInterceptor); interceptors.add(myInterceptor); sessionFactory.setPlugins(interceptors.toArray(new Interceptor[1]));
return sessionFactory.getObject(); } @Bean(name = "masterTransactionManager") public DataSourceTransactionManager masterTransactionManager(@Qualifier("masterDataSource") DataSource dataSource) { return new DataSourceTransactionManager(dataSource); } }
|
由于框架中使用多个数据源配置,因此使用数据库事务时,优先使用该事务时,需要在该方法上显著的添加@Primary注解
- 工具类:特殊字符转义
1 2 3 4 5 6 7 8 9 10 11 12 13
| import org.apache.commons.lang3.StringUtils;
public class EscapeUtil { public static String escapeChar(String before){ if(StringUtils.isNotBlank(before)){ before = before.replaceAll("\\\\", "\\\\\\\\"); before = before.replaceAll("_", "\\\\_"); before = before.replaceAll("%", "\\\\%"); } return before ; } }
|
重点关注
以上方法在关键字中包含有\可能会失效,失效的原因是由于查询的关键字的数据库字段排序规则为utf8_unicode_ci,如下图

要想不失效,查询的关键字的排序规则必须为utf8_general_ci,如下图

或者统一全部数据库字符集与排序规则分别为:utf8mb4与utf8mb4_general_ci,如下图

关注Github:1/2极客
关注博客:御前提笔小书童
关注网站:HuMingfeng
关注公众号:开发者的花花世界
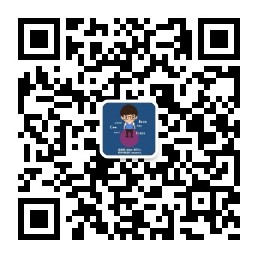
本作品采用知识共享署名 4.0 中国大陆许可协议进行许可,欢迎转载,但转载请注明来自御前提笔小书童,并保持转载后文章内容的完整。本人保留所有版权相关权利。
本文链接:https://royalscholar.cn/2019/11/30/MyBatis Plus之like模糊查询中包含有特殊字符/